User Attribute Transformation
In this comprehensive technical guide, we will explore how to leverage the User Attribute Transformation tab in 1Kosmos to customize user attributes and enhance the efficiency of your authentication processes.
Introduction
1Kosmos provides administrators with powerful capabilities to transform user attributes before sending them out in an assertion. This feature is particularly useful for concatenating user attributes, parsing multiple phone numbers from a single AD attribute, filtering out sensitive AD groups, and facilitating required transformations for application login.
Prerequisites
Before you begin, ensure the following:
- Your system must be on the latest version of AD Broker 1.08.01 or later.
- You have the permission to access the User Attribute Transformation tab.
This script supports only ECMA Script 5.1. ECMA Script 6 is not supported.
Accessing the User Attribute Transformation Tab
- Log in to your 1Kosmos admin console.
- Navigate to the Directory section.
- Click on the directory you want to manage.
- Locate the User Attribute Transformation tab, adjacent to the Advanced Configuration tab.
Using the User Attribute Transformation Tab
Once you've accessed the tab, you can perform the following tasks:
- Compose Scripts: In the JavaScript editor provided under the tab, you can compose scripts to transform user attributes according to your requirements.
- Save Script: To save the script, you must input an OTP (one-time password) for additional verification.
- Test Script: You can test the script by clicking the Test User Attributes button, entering a username, and viewing all associated user attributes if the username is valid.
Sample Use Cases and Scripts
Concatenate User Attributes
Combine multiple user attributes into a single attribute.
function transformUsers(usersStr) {
const staticValue = "Himank"; // static value to concatenate.
try {
const users = JSON.parse(usersStr);
for (const e of users) {
e.fname_lname = concatenateWithDistinctAttributes(e.givenname, e.lastName); //`e.lastname` is the reference to attribute configured in AD in AdminX.
e.static_value = concatenateWithStaticAttributes(staticValue, e.givenname); //`e.givenname` is the reference to attribute configured in AD in AdminX.
}
return JSON.stringify(users);
} catch (error) {
return {error}
}
}
function concatenateWithDistinctAttributes(attribute1, attribute2) { //concatenates two dynamic values configured in the AD(Active directory)
return `${attribute1}+${attribute2}`;
}
function concatenateWithStaticAttributes(staticValue, attribute) { //concatenates a dynamic and static values.
return `${staticValue}+${attribute}`;
}
Before Transformation:
Given Name: John
Last Name: Doe
After Transformation:
Concatenated Name: JohnDoe
Static Value Concatenated: HimankJohn
Split Mobiles and Landlines from Single AD Attributes
Split a single AD attribute containing phone numbers into separate values for landlines and mobiles.
function transformUsers(usersStr) {
try {
const users = JSON.parse(usersStr)
for (const e of users) {
if (e.facsimiletelephonenumber) {
const phones = parsePhoneString(e.facsimiletelephonenumber);
e.mobiles = phones.mobiles;
e.landlines = phones.landlines;
}
}
return JSON.stringify(users);
} catch (e) {
console.log(e.toString());
console.log(e.stack);
}
return usersStr;
}
function parsePhoneString(phoneString) {
const phones = phoneString.split(';');
const result = {
mobiles: [],
landlines: []
};
phones.forEach(function (phone) {
const match = phone.match(/([ML])(\d+)(x\d+)?/);
if (match) {
const [, type, number, extension] = match;
const phoneNumber = extension ? `${number}${extension}` : number;
if (type === 'M') {
result.mobiles.push(phoneNumber);
} else if (type === 'L') {
result.landlines.push(phoneNumber);
}
}
});
return result;
}
-
Before Attribute Transformation:
M:192837496478; M: 17839978923; L:2345672349 x728
-
After Attribute Transformation:
Mobiles: {192837496478, 17839978923} Landlines: {2345672349 x728}
UAC Parsing for Account Locked and Disabled
The following javascript verifies and returns the status of a user checking against the UAC values of the user.
function transformUsers(usersStr) {
try {
const users = JSON.parse(usersStr)
for (const e of users) {
const userAccountControlValue = e.useraccountcontrol || e.userAccountControl;
e.isUserDisabled = (userAccountControlValue & 0x0002) !== 0; // Checks the user attribute values in the AD and matches with the defined condition. Please note that ['512', '544', '66048', '262656', '1049088', '520']are hardcoded as UAC values for an active user.
e.isUserLocked = (e.lockouttime && e.lockouttime > 0) ? true : false;// A non-zero value of AD attribute `lockoutTime` denotes that the user is locked.
}
return JSON.stringify(users);
} catch (e) {
console.log(e.toString());
console.log(e.stack);
}
return usersStr;
}
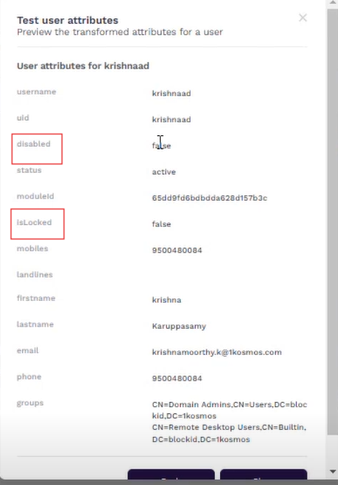
Conclusion
By leveraging the User Attribute Transformation tab in 1Kosmos, administrators can customize user attributes to meet specific requirements, enhance authentication processes, and improve overall system efficiency. Experiment with the provided scripts and unleash the full potential of user attribute transformation in 1Kosmos!